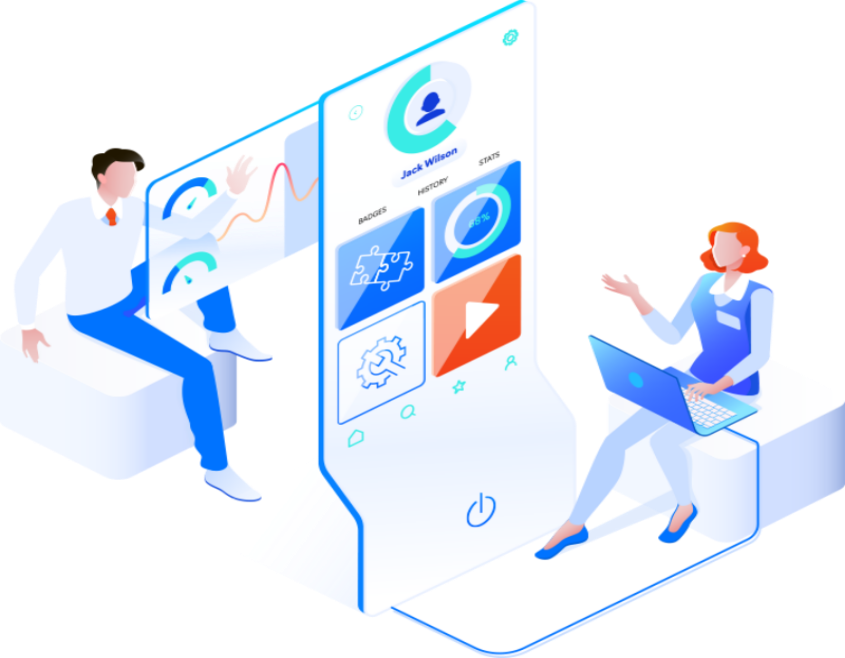

Introduction :
Ecommerce store owners understand the importance of marketing and developing a strong web presence. They create beautiful storefronts, invest in advertising campaigns, and build email marketing sequences to attract and win over customers.
Here we will be giving you a heads-up on how to create an ecommerce website for your business using Laravel.

First let’s look at how to install Laravel, and the requirements needed to get it up in running in the system:
Installation requirements
- Install the composer latest version and install Xampp in the working system
- Next get a text editor like Microsoft Vs code, Sublime text editor or notepad etc to edit your project code
- Download text editors from these URL’s
https://code.visualstudio.com/download
https://www.sublimetext.com/3 .
- Then select the folder directory in xampp and go into the htdocs folder then open in the command prompt
- Next step is to type in “composer global require laravel/installer” then hit enter, you can see that “laravel new example-app” ; “cd example-app” and “php artisan serve” shall start to run
- ● Once the app is installed , you can open the project folder via vscode or any text editor and you are ready to edit and create your own ecommerce project
Basic Ecommerce Features:
- A home page lists all products
- A product description page
- A checkout page to provide address details and payment information
- A cart page for as many products customer will select
- A dashboard for vendors to add/delete/update the product information and check sales, order and shipment details
- A customer dashboard to check the product purchased and shipment status
Benefits of Ecommerce site make using Laravel :
- Laravel is cost-friendly
- Laravel is scalable
- Laravel is easy to test
- Laravel offers a manageable shopping cart
- Laravel offers highly optimized e-commerce experience
User Roles Needed :
- Admin: The Administrator of the site
- Buyer: The customer who purchases goods from the site
- Vendors : The seller who sells the products on the site
Database connection:
- First we create a database in the mqsql with the name ecommerce, it will be connected to our Laravel project
- In the project find the .env file, on that file we change some lines like below
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=ecomm
DB_USERNAME=root
DB_PASSWORD=
DB_CONNECTION=”Database name”
DB_HOST=”Database host”
DB_PORT=”Database port”
DB_DATABASE=”Table name”
DB_USERNAME=”your username in database”
DB_PASSWORD=”Your password” .
Create Models:
- Model in MVC architecture holds business logic allows you to interact with the database. It provides the use of functions to perform a basic operation with our database tables
- Create model in the terminal by the command “$ php artisan make: model model name”
- By this command we make some models like product and user for our products and the users “$ php artisan make:model Product”&”$ php artisan make:model User”
Create Migrations:
- Migrations are like version control of your database schema in Laravel. It allows you to easily create and manage the database.
- Create model in the terminal by the command “$ php artisan make:migration create_users_table” and “$ php artisan make:migration create_products_table”
Create Seeders:
- Laravel created seed classes to push test data into our database
- Create seeder by “$ php artisan make:seed table-name” for your project “$php artisan make:seed Userstable” and “$php artisan make:seed Productstable”
- By the seeding in products table we giving the value in all columns and give all datas to the user table also
Create view pages:
- Create a registration page with a form like user name, email and password with some design using bootstrap or others
- Then in the registration page we make a login page with a form that contains email and password and a login button
- And make a home page with some designs of your choice for the products, this display view can be seen after the login
Create Routes :
- Create some Routes for your pages like login registration, home page and some extra pages for futures in routes we define url.
- Creating routes can be done by a syntax – Route::(‘method’)(‘/url’,[‘controller-name’::class,’function-name’]);
Route::post(“/register”, [UserController::class,’register’]);
Route::post(“/login”, [UserController::class,’login’]);
- For your project create login route by Route::post(‘/login’,[UserController::class,’login’]); similar for registration and the home pages also
Create Controllers:
- Create controllers by the command in terminal is “$ php artisan make:controller controller-name”
- Make some controllers for your project by using this command like $ php artisan make:controller UserController and $ php artisan make:controller ProductController
- These controllers are working based on the MVC pattern
Implementing Controllers :
- After creating the controllers and routes, we should connect the routes to the controllers like below.
Route::post(“/login”, [UserController::class,’login’]);
- The connection will be made with a function, and that is made in the controller.
- This is how the function is made.
class UserController extends Controller
{
//
function login(Request $req)
{“functionality code”}}
- By this way you can create functions for registration, login and home page .
- Here are few screen shots to help you understand how the function for registration looks like (Login and the Home page)
class ProductController extends Controller
{
//
function index()
{
“functionality code”
}}
- In top of this controller page we type model on top, as shown in the below snap shot because controller couldn’t find the model so we use this.
use App\Models\Product;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\DB;
- This way we implement the controller in the web.php routes page
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\UserController;
use App\Http\Controllers\ProductController;
Login Functionality
- We login via some methods like use session , Jetstream ,breeze etc
- In your project use browser’s session to get the data from the user it will be saved on the local session storage and check user input data using controller with our database then proceed to login
function login(Request $req)
{
$user = User::where([’email’=>$req->email])->first();
if (!$user || !Hash::check($req->password, $user->password))
{
return “Username or password is not matched”;
}
else{
$req->session()->put(‘user’,$user);
return redirect(‘/’);
}
}
Registration
- For registration create a route with post method and function for a new user in the database
- In this register function write some queries for saving user data to our database in user’s tables. And for password encryption we use hash encryption method so it is use in top of the User controller page like below.
use App\Models\User;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Hash;
Register Function :
function register(Request $req)
{
$user = new User;
$user->name=$req->name;
$user->email=$req->email;
$user->password=Hash::make($req->password);
$user->save();
return redirect(‘/login’);
}
Home page
- For your home page first seeding some product details to the database and make a view, then create a route and a controller to view it in the home page
- And home page contains login button and register button before login,
then you can see the cart and the order list this working after the user logs in - Our home page route will look like the one below
Route::get(“/”, [ProductController::class,’index’]);
- This is the function in the product controller, it will pull up all data from the database
function index()
{
$products=Product::all();
return view(‘product’,compact(‘products’));
}
- As we declare $products variable in product controller then it will be used in the blade view page like below
<div class=”carousel-inner”>
@foreach($products as $item)
<a href=”detail/{{$item[‘id’]}}”>
<div class=”carousel-item {{$item[‘id’]==1?’active’:”}} “>
<img src=”{{$item[‘gallery’]}}” class=” custom-slider mx-5 px-5″ >
<div class=”carousel-caption d-none d-md-block text-dark”>
<h5>{{$item[‘name’]}}</h5>
<p>{{$item[‘description’]}}</p>
</div>
</a>
</div>
@endforeach
</div>
Routes :
Routes we used in the project –
Route::post(“/login”, [UserController::class,’login’]);
Route::get(“/”, [ProductController::class,’index’]);
Route::get(“/detail/{id}”, [ProductController::class,’detail’]);
Route::get(“/search”, [ProductController::class,’search’]);
Route::post(“/add_to_cart”, [ProductController::class,’addToCart’]);
Route::get(“/cartlist”, [ProductController::class,’cartList’]);
Route::get(“/removecart/{id}”, [ProductController::class,’removeCart’]);
Route::get(“/ordernow”, [ProductController::class,’orderNow’]);
Route::post(“/orderplace”, [ProductController::class,’orderPlace’]);
Route::get(“/myorders”, [ProductController::class,’myOrders’]);
Route::view(“/register”, ‘register’);
Route::post(“/register”, [UserController::class,’register’]);
Route::post(“/login”, [UserController::class,’login’]);
we can make function in the controller for these routes and the view pages by the above method
After defining the routes and the controllers in the project then we will be designing the front with the blade.php file with bootstrap and also use Angular JS, Vue JS like this JavaScript frame works to build the core front end with that we can create separate dashboard for admin, users and vendors also, with separate authentication for each.
For the checkout process we can use any one of the payment processors like PayPal, Razor pay, Stripe etc. which can provide integration into the JavaScript and php frame work. .After the completion of front end and back end process, run the below command in terminal to run your application.
$ php artisan serve
Conclusion:
The above mentioned step by step procedure helps you to create, understand and build your own Ecommerce website using Laravel. Laravel gives you a user friendly experience and also is in your budget.
In case of any clarifications reach out to us in the comment section below.